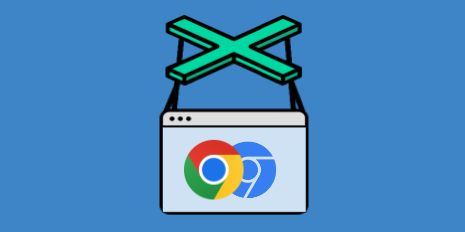
“Puppeteer” is a special tool made by the Chrome team that lets you use a web browser, like Chrome, without seeing it on your screen. You can tell it what to do using code. People use Puppeteer for things like copying information from websites, filling out online forms automatically, making PDF files, and lots of other tasks on the internet. It’s like having a robot that can browse the web for you.
Update your system
sudo apt-get update -y
Install dependencies
sudo apt install -y gconf-service libasound2 libatk1.0-0 libc6 libcairo2 libcups2 libdbus-1-3 libexpat1 libfontconfig1 libgcc1 libgconf-2-4 libgdk-pixbuf2.0-0 libglib2.0-0 libgtk-3-0 libnspr4 libpango-1.0-0 libpangocairo-1.0-0 libstdc++6 libx11-6 libx11-xcb1 libxcb1 libxcomposite1 libxcursor1 libxdamage1 libxext6 libxfixes3 libxi6 libxrandr2 libxrender1 libxss1 libxtst6 ca-certificates fonts-liberation libappindicator1 libnss3 lsb-release xdg-utils wget
Puppeteer, even though it doesn’t have a graphical interface, relies on some libraries to work with the X11 server. One of these libraries is libxcb, which needs a distributed library called libX11-xcb.so.1. To fix this, you can install the libx11-xcb1 package on Debian-based systems.
But sometimes, when you install a missing library, you might run into another missing library, and this can keep happening. That’s why it’s important to install a list of the required libraries to ensure Puppeteer runs smoothly.
Install NodeJS using NVM
$ wget https://raw.githubusercontent.com/nvm-sh/nvm/master/install.sh
$ bash install.sh
$ source ~/.bashrc
$ nvm list-remote
$ nvm install v18
$ nvm install node
$ nvm use 18
$ node --version
Install puppeteer
$ mkdir your-project
$ mkdir -p /home/ubuntu/.cache/puppeteer
$ chmod -R 700 /home/ubuntu/.cache/puppeteer
$ cd your-project
$ npm i
$ npm install puppeteer
$ cd /home/ubuntu/.cache/puppeteer
$ npm i
$ npm install puppeteer
Install Google Chrome
$ wget https://dl.google.com/linux/direct/google-chrome-stable_current_amd64.deb
$ sudo apt install ./google-chrome-stable_current_amd64.deb -y
$ google-chrome --version
To test if Puppeteer was installed successfully, you can create a JavaScript script named “test.js” and use the following code:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({
args: ["--no-sandbox", "--disable-setuid-sandbox"]
});
const page = await browser.newPage();
const url = 'https://google.com';
await page.goto(url);
await page.pdf({ path: 'page.pdf', format: 'A4' });
await browser.close();
})();
Test it:
node test.js
Troubleshooting :
Error 1 : Could not find Chrome (ver. xxx.xxx.xx).
1. you did not perform an installation before running the script (e.g. `npm install`) or
2. your cache path is incorrectly configured (which is: /home/ubuntu/.cache/puppeteer), please check chrome directory exist or not .
$ ls /home/ubuntu/.cache/puppeteer
3. Please verify whether Google Chrome is correctly installed
$ ls /usr/bin/google-chrome
Error 2: Failed to launch the browser process! [1003/185100.101396:ERROR:zygote_host_impl_linux.cc(100)] Running as root without –no-sandbox is not supported.
To fix the above (Running as root without --no-sandbox is not supported) error, use following steps:
Edit the below file
$ vim /usr/bin/google-chrome
instead of this line
exec -a "$0" "$HERE/chrome" "$@"
use line
exec -a "$0" "$HERE/chrome" "$@" --no-sandbox
Chrome Supported Version :
Chrome 117.0.5938.149 - Puppeteer v21.3.7
Chrome 117.0.5938.92 - Puppeteer v21.3.2
Chrome 117.0.5938.62 - Puppeteer v21.3.0
Chrome 116.0.5845.96 - Puppeteer v21.1.0
Chrome 115.0.5790.170 - Puppeteer v21.0.2
Chrome 115.0.5790.102 - Puppeteer v21.0.0
Chrome 115.0.5790.98 - Puppeteer v20.9.0
Chrome 114.0.5735.133 - Puppeteer v20.7.2
Chrome 114.0.5735.90 - Puppeteer v20.6.0
Chrome 113.0.5672.63 - Puppeteer v20.1.0
Chrome 112.0.5615.121 - Puppeteer v20.0.0