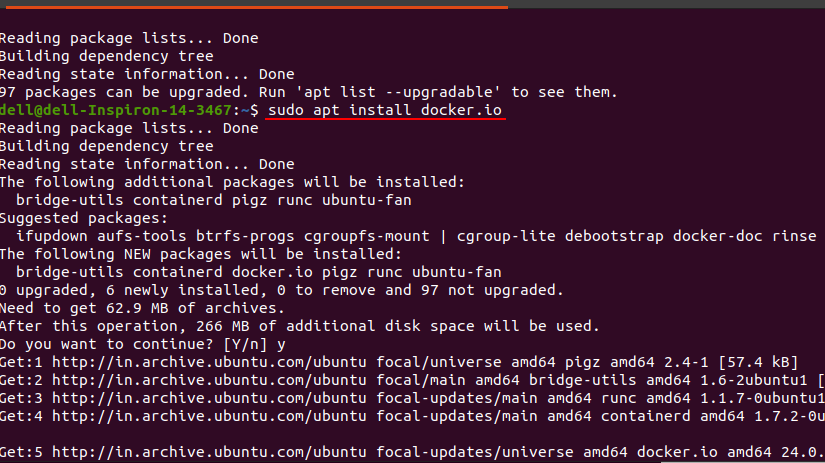
Component of docker architecture
Docker uses a client-server architecture, which allows you to interact with Docker through a command-line interface (CLI) or a graphical user interface (GUI) while the Docker daemon (server) does the actual work of managing containers. Here’s an overview of the Docker architecture:
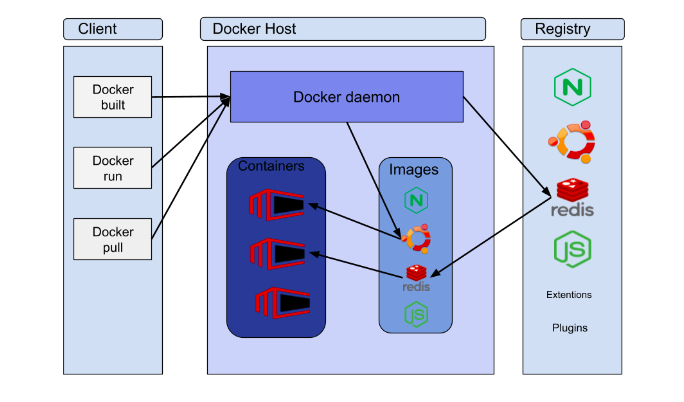
Docker Client
This is the interface that a user interacts with. It’s usually a command-line tool (CLI) or a GUI. When you issue a command like “run a container,” the Docker Client translates it into a request to the Docker Daemon.
Docker Daemon
The Docker Daemon is a background process running on your system. It’s responsible for the heavy lifting of container management. It listens for API requests from the Docker Client and acts on them. It can create, run, and manage containers, as well as handle tasks like image management, containerizing, data volumes, and network configuration.
Docker Registry
Docker images, which are important snapshots of applications and their dependencies, are stored in Docker Registries. These are like online repositories where you can upload, share, and download container images. Docker Hub is a popular public registry, but you can also have private ones for your organization.
Default Registry: Docker comes with a default registry called the Docker Hub. It’s like a public library that contains a vast collection of images for various programming languages and platforms. When you request an image in Docker, it first checks the Docker Hub to see if it’s available there. This is like going to a public library to find a book.
Private Registry: Just as you can have your personal book collection at home, you can also have your private Docker registry. This is like having your own library where you store images that are specific to your needs, like custom applications or configurations. You can configure
commands:
to log to the docker hub,
docker login
Username: Password:
Docker Images
Images in Docker serve as templates or snapshots of a file system with a specific set of files and configurations.
Application Dependencies: Images specify all the libraries, binaries, and resources required for an application to run. This includes everything from the base operating system to any additional software packages or libraries that an application needs to run.
Launch Processes: Images define the initial command or process that should be executed when a container is started. This command often kicks off the main application or service within the container.
Commands
To see all the images present in your local machine run
docker images
To find out images in Docker Hub.
docker search <image_name>
To download/pull Images from Docker Hub to your local machine.
docker pull <image_name>
To push a Docker image to a registry i.e. Docker Hub
docker push <image_name>:<tag>
To add or change tags for a Docker image
docker tag <source_image>[:<source_tag>] <target_image>[:<target_tag>]
- <source_image>- image id of that image which you want to tag.
- :<source_tag>- If the source image has a tag, you can specify it here. If not leave it default to latest. used when you want to change the tag.
- <target_image>This is the new name you want to give to your image
- <target_tag>-tag you want to give to your image
To get detailed information about a Docker image, including its layers and metadata.
docker image inspect <image_name/id>:<tag>
To remove a Docker Image you can use.
docker rmi <image_name_or_id>
Docker Build
Docker Build is a core feature of Docker Engine used extensively in software development. At its core, it’s a command that facilitates the creation of Docker images. This process involves taking your application’s source code, along with necessary dependencies and configuration, and packaging it into a standalone, runnable image.
Docker begins with a Dockerfile.
Docker File
A Dockerfile is like a set of instructions written in plain text. It tells a computer what to do step by step to create a special kind of package called an “image.” This image can contain everything an application needs to run.
So, with a Dockerfile, you can automate the process of building this package. It’s like giving your computer a recipe to follow. It reads the Dockerfile and carries out each instruction to create the image you want. This makes it easier to create and manage these packages for your applications.
Here are the most common types of instructions used to build a Docker File,
FROM base_image:tag # A base image use to create the container
MAINTAINER="Your Name" # author/owner name
WORKDIR /app # Set the working directory inside the container where instruction will be executed
COPY source_path destination_path # Copy files from the host into the container
RUN apt-get update && apt-get install -y package1 package2 # Executes a command during the image build process. Commonly used for installing packages and dependencies.
EXPOSE port_number # Expose ports for the container (for runtime). Informs Docker that the container will listen on the specified port at runtime. It doesn't actually publish the port.
ENV VAR_NAME=value # Sets environment variables inside the container.
CMD ["executable", "arg1", "arg2"] # It defines the default command to run when a container is started. It can be overridden at runtime.only one CMD per Dockerfile.
VOLUME ["/data"] # Define a volume for data storage, it helps in creating a mount point and marks it for storing persistent data.
SHELL ["/bin/bash", "-c"] # Execute a shell command during build..
ENTRYPOINT ["executable", "arg1", "arg2"] # Set the entry point to a script. The ENTRYPOINT instruction specifies the command that should be run when the container starts. It is often used for specifying the main executable or entry point of your application.
Building images on your local machine
- let’s create a docker file to run Hello World
- the first step is to create a docker file in a particular folder named Dockerfie
- Edit the docker file and write a code to build an image
FROM ubuntu
MAINTAINER <owner_name>
RUN apt-get update
CMD ["echo","Hello World"]
build your image from the docker file using the below command
#when you are present in tne same directory where dockerfile present
docker build <image_name>:<tag> .
#when you are in different directory yauneed to specify thepath of your dockerfile
docker build /path/to/dockerfile/ <image_name>:<tag>
Use the docker images command to see the image that you build. you will find your image with the name that you give and a unique Image ID.
Containers
In Docker, containers are lightweight, self-contained packages for running software. They include everything an application needs, such as code, libraries, and settings. Act as an executable instance of Docker images.
Images: Docker images are like blueprints that contain all the instructions for creating a container. They include the application code, dependencies, and configuration.
Containers: Containers are the actual running instances of Docker images. When you start a Docker container, you’re essentially using an image to create a live environment to run your application. Each container is isolated from others, so they don’t interfere with each other.
Isolation: Docker containers are isolated from each other, much like how objects in code are encapsulated and don’t affect each other’s data.
Commands
To show all the docker processes actively running containers “ps stands for process status”,
docker ps
To see the all containers running in the background
docker ps -a
This command is used to run a container with the help of an image.
docker run --name <container_name> <image_id/name>
This command is used to view detailed information about a container.
docker inspect <container_name/id>
Start containers from an image
–name defines the name of the containers
-d define container to run in detach mode i.e. running in the background
-it defines the continuous running of the containers
docker run --name <container_name> -it -d <imag_ID/Name>
Start, Stop, and restart Containers:
docker start <container_ID/Name>
docker stop <container_ID/Name>
docker restart <container_ID>
Runs a command inside a running container.
To go inside the container.
docker exec -it <container_ID> <command>
docker attach <container_ID>
To view details of containers
docker inspect <container_ID>
To remove/delete container.
-f for forcefully removing the container
Removes all stopped containers, freeing up disk space
docker rm <container_ID>
docker container prune
to display the logs of the container,
docker logs <container_ID>
Copies files from your local machine to a container, or vice versa.
docker cp <local_path> <container_ID>:<container_path>
To create a volume to store data, volume is basically used to store the data outside the container
-v used to specify a volume to be mounted to the container
docker run -v <volume_name>:<container_path> <image>
Shows real-time resource usage statistics of a container.
docker stats <container_ID>
Registries
A registry is a library or a storage place for images, which are the building blocks of containers.
Default Registry: Docker comes with a default registry called the Docker Hub. It’s like a public library that contains a vast collection of images for various programming languages and platforms. When you request an image in Docker, it first checks the Docker Hub to see if it’s available there. This is like going to a public library to find a book.
Private Registry: Just as you can have your personal book collection at home, you can also have your private Docker registry. This is like having your own library where you store images that are specific to your needs, like custom applications or configurations. You can configure
Docker engine
The Docker Engine is like the heart of Docker, where everything happens. It’s the software that makes containers work on your computer.
Docker Daemon: It runs on your computer and does all the heavy lifting. It’s responsible for creating, managing, and running containers.
Docker Client: It’s a command-line tool you use to tell the Docker Daemon what to do. You give it special commands, and it communicates with the Daemon to make things happen.
Docker compose
Docker Compose is a valuable tool for defining and running multi-container Docker applications. It simplifies the process of configuring and managing complex applications by utilizing a YAML file to specify the setup of your application’s various services. This configuration file acts as a blueprint, and with a single command, you can create and launch all the services according to the defined configuration.
The significant benefit of using Docker Compose is that you can specify your application’s entire setup in a single file, which you keep at the root of your project’s repository. This file can be version-controlled, making it easy for others to collaborate on your project. All someone needs to do is clone your repository and start the application using Docker Compose. As a result, you may find many projects on platforms like GitHub and GitLab adopting this approach.
command to install docker on Ubuntu
sudo apt install docker-compose
To check the version of the docker
docker-compose --version
Docker-compose.yml File
Docker Compose is the tool we use for setting up our local development environments. To define how the containers work together, Docker Compose relies on a file called docker-compose.yml
. This file specifies important details, such as which images are needed, which ports should be made available, whether the containers can access the host’s files, and what commands to execute when they begin running. In essence, it’s a blueprint that describes how everything should work together.
Running docker-compose
docker-compose up -d
To see running container and their state with ports.
docker-compose ps
To check the logs of the docker-compose use the command:
docker-compose logs
To stop, pause, and unpause the running docker-compose:
docker-compose stop
docker-compose pause
docker-compose unpause
To remove the containers, networks, and volumes associated with this containerized environment, use this command:
docker-compose down